Casino Siteleri (güncel liste)
Canlı casino siteleri sizlere online olarak gerçek casino deneyimini yaşatmayı arzulayan ve hedefleyen sitelerdir. Bu siteler dağıttıkları freespin ve slot iadesi, kayıp bonusu, casino hoşgeldin bonusu gibi bonuslarla öne çıkar ve bu şekilde sınıflandırılır. Bu siteler 2023 yılında olduğu gibi 2024 yılında da spin, slot ve konularında popülerliğini sürdürecektir.
Online sitelerin çoğu yatırım şartsız olarak hizmet vermektedir. Kayıt olduktan sonra otomatik olarak bakiye deneme bonusu adı altında hesaba yatmaktadır.
Kıbrısta bulunan casinolarda birçok slot ve blackjack oyunu oynatılmaktadır. Bu firma ve casinolar içerisinde birçok online hizmet veren casino mevcuttur. Diğer sitelere göre daha fazla tercih edilmektedir.
Bir çok insan gerçek casino ile casino sitelerinin arasında dağlar kadar fark olduğu söyler. Ancak bize göre online casino siteleri daha avantajlıdır. Bunun birden fazla sebebi var başlıca sebeplerinden birisi gerçek casino da ne zaman duracağınızı bilemeyebilirsiniz, online casino da ise sadece çıkış yaparak oyunu durdurabilirsiniz.
En iyi casino siteleri;
- Restbet
- Meritroyal
- Onwin
- İkimisli
- Grandpasha
- 1xbet
- Youwin casino
Güvenilir Casino Siteleri Nasıl Seçilmeli ?
Güvenilir bir şekilde hizmet alabileceğiniz, kazançlarını ise sorun yaşamadan çekebileceğiniz siteleri tercih etmeniz gerekmektedir. Güvenilir casino siteleri, lisanslı bir şekilde hizmet vermektedir. Güvenilir ödeme yöntemleri üzerinden oyuncularına kazançlarını vermektedir. Bu şirketler sadece Türkiye’de değil. Aynı zamanda global ölçekte hizmet vermektedir. Güvenilir casino siteleri nasıl anlaşılır? hakkında detaylı bilgi verilecektir.
2024 yılına girerken sektörün en güvenilir siteler editör kadromuz tarafından listelenmiştir. Listemizde paylaşılan online kumar siteleri üzerinden hizmet alın. Ayrıca butonlar üzerinden hesabınızı oluşturmanız durumunda deneme bonusu ile oynamaya başlayın.
Güvenilir casino siteleri müşterilerini mağdur etmeyen, çekimleri hızlı gönderen, müşteri hizmetlerinde güler yüzlü pozitif destek veren siteler olarak nitelendirilebilir. Şunu belirtmekte fayda var bugün bir çok güvenilir sitenin şikayetlerini ele aldığımızda kullanıcılar paralarını çekemediklerinden bahsediyor.
Bu tip şikayetlerin ardı arkası görülmeyecek kadar çok olabilir. İlk başta akıllara gelen site güvenilmez gibisinden olsa da sitenin gerçek durumuna bakıldığında kullanıcıların bonus aldığı ve o bonusun çevrim şartına uyulmadığı anlaşılır. Bu tip şikayetlerden ziyade bir sitenin kaç senedir aktif olduğu, canlı yorumlar ele alınabilir. Aynı zaman da bonus almadan önce çevrim şartlarını iyice incelemeniz gerekir.
Canlı Siteler (2024 tahminleri)
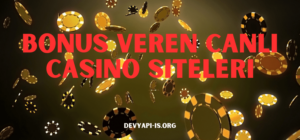
Bonus Veren Canlı Casino Siteleri
Bulundurdukları kurpiyerler aracılığı ile canlı kumar oynatan sitelere denir. Yalnız bir noktaya dikkat etmek gerekiyor her site de aradığınız oyunu bulamayabilirsiniz. Oynamak istediğiniz oyunun adını ilgili siteye üye olmadan önce demo oyunlar kısmında inceleyebilir ve görebilirsiniz. Türkiye den giriş yaparken BTK engeli yüzünden zor anlar yaşayabilirsiniz. Bununla ilgili sitemizde bulunan tablodan güncel adres e giriş yapabilir veya VPN indirerek yeni adresi bulabilirsiniz. Keno, blackjack, rulet, poker, canlı okey oynatan sitelerdir.
2024 yılında da canlı casino sitelerinde ortalama olarak 200 tl bonus verecektir. Sadece casino oyunlarından değil tüm bahis oyunlarında mevcut olacaktır. Birçok websitesinde yatırım şartı olmadan oyunlar oynanabilecektir. Oyuncu sayısı 2024 yılında artış gösterecektir.
Yasal Siteler Var Mı?
Yasal siteleri henüz Türkiye de faaliyet göstermeyen sitelerdir. Sadece iddaa üzerine faaliyet gösteren sayılı siteler olsa da verdikleri oranlar çok düşük olduğu için kullanıcılar her zaman canlı bahis sitelerini tercih etmektedir.
İlk olarak bilmeniz geren illegal siteleri veya kaçak bahis siteleri kötü siteler değillerdir. Bu sitelerin tek ortak bir amacı bulunuyor müşterilerini memnun etmek. Bunu başaramayan siteler zaten kapatılıyor veya batıyorlar, başarabilenler ise yıllardır açık kalıyor. Yani siz değerli ziyaretçilerimiz canlı casino siteleri sizin için çalışıyor, memnun edilecek biri varsa oda sizlersiniz.
Sitelerinin adres değişikliği üzerinden hizmet vermesinin nedeni yasal olarak hizmet verememesinden kaynaklanmaktadır. BTK tarafından web sayfaları engellendiğinden dolayı adres yenilerek hizmetlerine devam etmektedir. Güncel adresleri kullanarak sizlerde canlı casino ve slot oyunları oynayabilirsiniz.
Hangi Oyunlar Var?
Geniş bir oyun hizmeti bulunmaktadır. Kumarhanelerde oynanan poker blackjack, rulet ve slot oyunları başta olmak üzere yüzlerce farklı casino oyunları bulunmaktadır. Tüm kumar oyunları oynanabilmektedir. Spor bahisler, E-spor bahisleri, tombala başta olmak üzere şans oyunları da sunmaktadır. Aynı zamanda bahis siteleri olarak da geçmektedir.

Deneme Bonusu Veren Siteler
En fazla oynanan oyunlar şunlardır:
- Poker
- BlackJack
- Rulet
- Crazy Time
- Aviator
- Sweet Bonanza
- Gates of Olmypus
- Video slot oyunları
İnsanlar tarafından en fazla tercih edilen casino oyunlarıdır. Bu oyunlar dışında binlerce farklı oyun bulunmaktadır. Son olarak unutmayın ki casino sitelerindeki tüm oyunlar şans üzerine kurulu eğlenceli oyunlardır. Bundan dolayı bir kazanç kapısından ziyade eğlence dünyası olarak görülmelidir.
Para Yatırma ve Çekme Nasıl Yapılır?
Casino oyunlarında oyunlara dahil olmak için kayıt olmanız ve sonrasında para yatırmanız gerekmektedir. Gerçek paralar üzerinden işlem yapıldığından dolayı sitelerin güvenilir olması gerektiğinden bahsettik. Güvenilir casino siteleri aynı zamanda geniş bir ödeme yöntemi sunmaktadır. Oyuncular istedikleri yöntemi kullanarak hesaplarına para yatırabilmekte ve çekebilmektedir.
Sitelerde hesabınızı oluşturduktan sonra para yatırma bölümü bulunmaktadır. Para yatırma bölümüne tıklayın. Karşınıza işlemlerinizi yapabileceğiniz yöntemler çıkacaktır. İstediğiniz yöntemi seçtikten sonra işleminizi nasıl yapacağınız sizlere detaylı bir şekilde anlatılmaktadır. Aktarılan adımları takip ederek para yatırma işleminizi kolay bir şekilde yapın. İşleminiz en fazla 5 dk içinde gerçekleşmektedir.
Ödeme yöntemleri şunlardır:
- Havale / EFT
- Papara
- Cepbank
- Payfix
- Ecopay
- Pay-co
- Mobil Ödeme
- Kripto Paralar
- Jeton
- Kredi Kartı
Yöntemleri başta olmak üzere işlemlerinizi güvenilir bir şekilde yapın. Ayrıca aynı yöntemler üzerinden kazançlarınızı çekebilirsiniz. Güvenilir casino siteleri üzerinden kazançlarınızı sorun yaşamadan anında hesabınıza aktarın. Para çekme bölümüne giriş yaptıktan sonra karşınıza ödeme yöntemi çıkacaktır. Ödeme yönteminizi seçtikten sonra belirtilen yere adresinizi yazarak kazanıcını çekin. Çekim işleminizi verdikten sonra en fazla 15 dk içinde kazancınız gönderilmektedir.
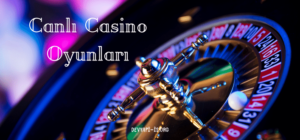
Canlı Casino Oyunları
Para Kazanma Yöntemleri
Casino kasa katılama, Casino oyunlarından nasıl kazanç sağlanır?. Kumar oyunları başta olmak üzere kumar oyunları oynayan insanların büyük bir bölümü yüksek kazançlar sağlama umudu ile oynamaktadır. Özellikle slot oyunları oyuncuların ilk durak yeri olmuştur. Çünkü slotlar küçük miktarlara bile yüksek kazançlar verebilmektedir.
Oyuncular nasıl kazanç sağlanır? Diye sormaktadır. Lakin oyunlar şans üzerine kuruludur. Ödeme alıp almamanız tamamen şanınızı bağlıdır. Kart oyunları oynuyorsanız eğer elinizi gelen kartlara göre kazanç sağlayabilirsiniz.
Canlı Casino Oyunları Oynanan Siteler
Kumarhanelerde kurpiyerlerin sunumlarıyla oynanan tüm kumar oyunları başta olmak üzere canlı bir şekilde oynayabileceğiniz yüzlerce farklı canlı casino oyunu bulunmaktadır. Poker, BlackJack, Rulet gibi popüler canlı oyunların yanında yüzlerce farklı içerik sizleri beklemekte. En önemlisi ise her şey canlı bir şekilde oyunculara aktarılmaktadır. Masalarda olan her şey oyunculara şeffaf bir şekilde aktarılmaktadır. Ayrıca oyunların tamamı kurpiyerler tarafından sunulmaktadır.
Gerçek kumarhanelerden hizmet alın. Canlı casino siteleri sayesinde artık internete bağlı olduğunuz her yerden kumarhanelere ulaşarak oyunlara dahil olabilirsiniz. Sitelerde hesabınızı oluşturduktan sonra canlı casino bölümüne girerek istediğiniz oyunlarda şansınızı deneyebilirsiniz. Canlı olarak aktarılan oyunların hepsi kurpiyerler tarafından sunulmaktadır. Ayrıca oyunlarda bulunan herkes sizler gibi gerçek oyuncudur.
Artık kumar oyunları oynamak için Kıbrıs, Batum kumarhanelerinize gitmenize gerek yok. Tüm hizmetlere internet üzerinden ulaşın. Tek fark sanal olmasıdır. Oyuncular gerçek bir hizmete anında ulaşabilmektedir. İster mobil cihazlarınızdan isterse bilgisayarınızdan, internete bağlı olduğunuz her an kumar oyunları oynayın.
En İyi Casino Slot Siteleri Listesi
Kumar severler tarafından en fazla tercih edilen oyunların başında slotlar gelmektedir. Kumarhanelerde makineler üzerinden oynanan oyunlar slotlardır. Tamamen şans üzerinden kurulu, eğlenceli ve tek bir spinde epik kazançlar elde edebileceğiniz oyunlardır. Kumarhanelerdeki slotlar, klasik slot oyunları olarak aktarılmaktadır.
Online kumarhanelerde ise slot oyunlarında jackpot kazancını öğrenmek için her siteyi ayrı ayrı kontrol edebilirsiniz. Bir site de yakın zamanda mega kazanç elde edilmiş ise o sitede rakam düşük olacaktır.
Her site de değişen jackpot miktarları olur, bu miktarlar sağlayıcılara göre ve site anlaşmalarına göre değişir. Evulation en çok jackpot kazancı sağlayan sağlayıcılardan birisidir.
Alan adresimiz 2024 sezonu için www.mockobjects.com olarak güncellenmiştir. Unutmayın ki kumar sürekli para elde edebileceğiniz kazanç kapısı değil, aksine sürekli para kaybedebileceğiniz tehlikeli bir oyundur. Bu bağlamda kumar oynarken sınırlarınızı zorlamamayı ve durmayı bilmenizi öneriyoruz.